Step 1 Generate Printing Data: Template Printing Function
The template printing function generates printing data by combining a template, which defines a print layout, and field data, which replaces the text in a specified area in the template with a desired character string.
Separating data used for printing into a template and field data allows users to use data variable for each printing easily and reuse the print layout.
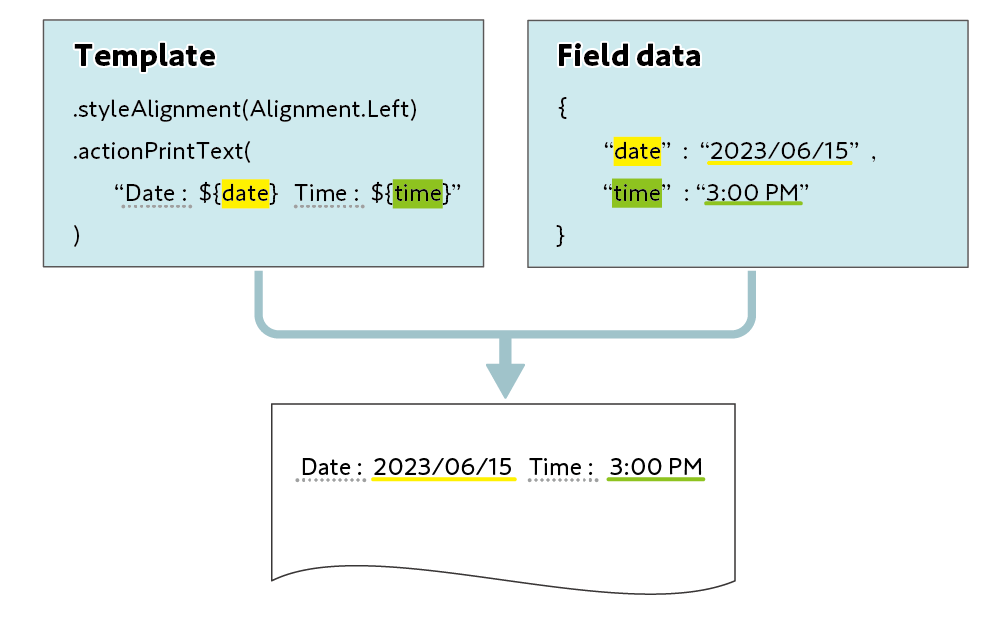
Four Major Useful Features of Template Printing Function
1. Specifying field data in JSON format
You can import different data for each printing, such as a receipt or label, in JSON format from the outside by methods such as Web API and use it as printing data.
2. Reusing a print layout
By saving fixed printing content as a template, you can easily reuse a print layout created previously.
You can modify the layout just by editing the template.
3. Support for array field data
Once you specify keys that represent array elements of field data in the template, you can automatically print all the elements in the array.
4. Flexible number format specifications
You can perform detailed operations, such as number conversion between integers and decimals and number printing with the number of digits specified, in a C language-like format.
Implement Printing Data Creation Process Using Template Function
The following image is a print sample using the template printing function.
To perform this printing using the template printing function, create a template using the procedure below (*) and prepare field data.
* The description of API calls not required to understand the template creation process is omitted.
The sample codes for a template and field data are available at the bottom of the page.
1 Generate the StarXpandCommandBuilder instance, which is used to create a template.
Call the addDocument method to add DocumentBuilder, and then call the addPrinter method to add PrinterBuilder.
Call the PrinterBuilder methods to create the template.
let builder = StarXpandCommand.StarXpandCommandBuilder() _ = builder.addDocument(StarXpandCommand.DocumentBuilder() .addPrinter(StarXpandCommand.PrinterBuilder()
2 To print the value of the store_name key (" Star Cafe "), call the actionPrintText method with a character string containing a replacing specifier as its argument.
.actionPrintText("${store_name}\n")
3 To print the order_number key value ("#2-007"), call the actionPrintText method by passing a character string containing replacing specifiers.
As shown in this case, normal texts and replacing specifiers can coexist.
In addition, because the printed number of characters is specified by the actionPrintText method, the TextParameter class setWidth method is called.
This fixes the print position and width of the character string, and can prevent disruption of the layout caused by an increase or decrease in the number of characters.
.actionPrintText( "Order ${order_number}", StarXpandCommand.Printer.TextParameter() .setWidth( 16 ) )
In order to prevent the disruption of the layout caused by a change in the number of field data characters, there is a function which uses the actionPrintText method parameter argument to specify in advance the number of characters to print.
For details of this function, refer to the TextParameter class setWidth method and the TextWidthParameter class API reference.
4 To print the field data of array elements, specify the template area to repeat.
First, call the setEnableArrayFieldData method of the TemplateExtensionParameter class by passing true as the argument.
Next, call the setTemplateExtension method of the PrinterParameter class by passing the TemplateExtensionParameter instance to the method.
Then, call the PrinterParameter instance by passing it to the PrinterBuilder constructor.
This API call for PrinterBuilder is repeated as many times as the number of array elements specified in Step 5.
.add( StarXpandCommand.PrinterBuilder( StarXpandCommand.Printer.PrinterParameter() .setTemplateExtension( StarXpandCommand.TemplateExtensionParameter() .setEnableArrayFieldData(true) ) )
5 To print the field data of array elements, call the actionPrintText method by passing a character string containing replacing specifiers that specify the field data of array elements (item_list key values).
.actionPrintText( "${item_list.quantity}", StarXpandCommand.Printer.TextParameter() .setWidth(2) ) .actionPrintText( "${item_list.name}", StarXpandCommand.Printer.TextParameter() .setWidth(24) ) .actionPrintText( "${item_list.unit_price%6.2lf}\n", StarXpandCommand.Printer.TextParameter() .setWidth(6) )
6 To print the numeric-type subtotal key value (8.24) in a specified format, call the actionPrintText method by passing a character string containing replacing specifiers that specify the number format.
.actionPrintText( "${subtotal%6.2lf}\n", StarXpandCommand.Printer.TextParameter() .setWidth( 6, StarXpandCommand.Printer.TextWidthParameter() .setEllipsizeType(.end) ) )
7 To print the barcode of the transaction_id key value ("0123456789"), call the actionPrintBarcode method by passing a character string containing a replacing specifier.
.actionPrintBarcode( StarXpandCommand.Printer.BarcodeParameter(content: "${transaction_id}", symbology: .code128) .setPrintHRI(true) )
8 To obtain the template, call the getCommands method.
val commands = builder.getCommands()
9 Prepare field data in JSON format. The texts in the specified areas in the template are replaced with this field data.
For how to send field data to a printer using the template created in this section, refer to Step 2.
Sample code
Field data
{
"store_name" : " Star Cafe ",
"order_number" : "#2-007",
"time" : "10/16 11:13PM",
"sales_type" : "Walk-in",
"server" : "Jane",
"transaction_id" : "0123456789",
"item_list" : [
{
"name" : "Vanilla Latte",
"unit_price" : 4.99,
"quantity" : 1
},
{
"name" : "Chocolate Chip Cookie",
"unit_price" : 3.25,
"quantity" : 1
}
],
"subtotal" : 8.24,
"tax" : 0.73,
"total" : 8.97,
"credit_card_number" : "VISA 0123",
"approval_code" : "OK2443",
"amount" : 8.97,
"address" : "123 Star Road, City,\nState 12345",
"tel" : "123-456-7890",
"mail" : "info@star-m.jp",
"url" : "star-m.jp"
}
Template Creation Process
let builder = StarXpandCommand.StarXpandCommandBuilder()
_ = builder.addDocument(StarXpandCommand.DocumentBuilder()
.settingPrintableArea(48.0)
.addPrinter(StarXpandCommand.PrinterBuilder()
.actionPrintImage(StarXpandCommand.Printer.ImageParameter(image: logo, width: 406))
.styleInternationalCharacter(.usa)
.styleCharacterSpace(0.0)
.add(
StarXpandCommand.PrinterBuilder()
.styleAlignment(.center)
.styleBold(true)
.styleInvert(true)
.styleMagnification(StarXpandCommand.MagnificationParameter(width: 2, height: 2))
.actionPrintText("${store_name}\n")
)
.actionFeed(1.0)
.actionPrintText(
"Order ${order_number}",
StarXpandCommand.Printer.TextParameter()
.setWidth(
16
)
)
.actionPrintText(" ")
.actionPrintText(
"${time}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
15,
StarXpandCommand.Printer.TextWidthParameter()
.setAlignment(.right)
.setEllipsizeType(.end)
)
)
.actionPrintText(
"Sale for ${sales_type}",
StarXpandCommand.Printer.TextParameter()
.setWidth(16)
)
.actionPrintText(" ")
.actionPrintText(
"Served by ${server}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
15,
StarXpandCommand.Printer.TextWidthParameter()
.setAlignment(.right)
.setEllipsizeType(.end)
)
)
.actionPrintText(
"Transaction #${transaction_id}\n"
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.add(
StarXpandCommand.PrinterBuilder(
StarXpandCommand.Printer.PrinterParameter()
.setTemplateExtension(
StarXpandCommand.TemplateExtensionParameter()
.setEnableArrayFieldData(true)
)
)
.actionPrintText(
"${item_list.quantity}",
StarXpandCommand.Printer.TextParameter()
.setWidth(2)
)
.actionPrintText(
"${item_list.name}",
StarXpandCommand.Printer.TextParameter()
.setWidth(24)
)
.actionPrintText(
"${item_list.unit_price%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(6)
)
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.actionPrintText(
"Subtotal",
StarXpandCommand.Printer.TextParameter()
.setWidth(26)
)
.actionPrintText(
"${subtotal%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
6,
StarXpandCommand.Printer.TextWidthParameter()
.setEllipsizeType(.end)
)
)
.actionPrintText(
"Tax",
StarXpandCommand.Printer.TextParameter()
.setWidth(26)
)
.actionPrintText(
"${tax%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
6,
StarXpandCommand.Printer.TextWidthParameter()
.setEllipsizeType(.end)
)
)
.add(
StarXpandCommand.PrinterBuilder()
.styleBold(true)
.actionPrintText(
"Total",
StarXpandCommand.Printer.TextParameter()
.setWidth(26)
)
.actionPrintText(
"${total%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
6,
StarXpandCommand.Printer.TextWidthParameter()
.setEllipsizeType(.end)
)
)
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.actionPrintText(
"${credit_card_number}",
StarXpandCommand.Printer.TextParameter()
.setWidth(26)
)
.actionPrintText(
"${total%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
6,
StarXpandCommand.Printer.TextWidthParameter()
.setEllipsizeType(.end)
)
)
.actionPrintText(
"Approval Code",
StarXpandCommand.Printer.TextParameter()
.setWidth(16)
)
.actionPrintText(
"${approval_code}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
16,
StarXpandCommand.Printer.TextWidthParameter()
.setAlignment(.right)
)
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.actionPrintText(
"Amount",
StarXpandCommand.Printer.TextParameter()
.setWidth(26)
)
.actionPrintText(
"${amount%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
6,
StarXpandCommand.Printer.TextWidthParameter()
.setEllipsizeType(.end)
)
)
.actionPrintText(
"Total",
StarXpandCommand.Printer.TextParameter()
.setWidth(26)
)
.actionPrintText(
"${total%6.2lf}\n",
StarXpandCommand.Printer.TextParameter()
.setWidth(
6,
StarXpandCommand.Printer.TextWidthParameter()
.setEllipsizeType(.end)
)
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.actionPrintText(
"Signature"
)
.add(
StarXpandCommand.PrinterBuilder()
.styleAlignment(.center)
.actionPrintImage(
StarXpandCommand.Printer.ImageParameter(image: signature, width: 256)
)
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width: 32.0)
.setX(8.0)
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.actionPrintText("\n")
.styleAlignment(.center)
.actionPrintText(
"${address}\n"
)
.actionPrintText(
"${tel}\n"
)
.actionPrintText(
"${mail}\n"
)
.actionFeed(1.0)
.actionPrintText(
"${url}\n"
)
.actionPrintRuledLine(
StarXpandCommand.Printer.RuledLineParameter(width:48.0)
)
.actionFeed(2.0)
.actionPrintText(
"Powered by Star Micronics\n"
)
.actionPrintBarcode(
StarXpandCommand.Printer.BarcodeParameter(content: "${transaction_id}", symbology: .code128)
.setPrintHRI(true)
)
.actionCut(.partial)
let command = builder.getCommands()
The following image is a print sample using the template printing function.
To perform this printing using the template printing function, create a template using the procedure below (*) and prepare field data.
* The description of API calls not required to understand the template creation process is omitted.
The sample codes for a template and field data are available at the bottom of the page.
1 Generate the StarXpandCommandBuilder instance, which is used to create a template.
Call the addDocument method to add DocumentBuilder, and then call the addPrinter method to add PrinterBuilder.
Call the PrinterBuilder methods to create the template.
val builder = StarXpandCommandBuilder() builder.addDocument( DocumentBuilder() .addPrinter( PrinterBuilder()
2 To print the value of the store_name key (" Star Cafe "), call the actionPrintText method with a character string containing a replacing specifier as its argument.
.actionPrintText("\${store_name}\n")
3 To print the order_number key value ("#2-007"), call the actionPrintText method by passing a character string containing replacing specifiers.
As shown in this case, normal texts and replacing specifiers can coexist.
In addition, because the printed number of characters is specified by the actionPrintText method,
the TextParameter class setWidth method is called.
This fixes the print position and width of the character string, and can prevent disruption of the layout caused by an increase or decrease in the number of characters.
.actionPrintText( "Order \${order_number}", TextParameter() .setWidth( 16 ) )
In order to prevent the disruption of the layout caused by a change in the number of field data characters, there is a function which uses the actionPrintText method parameter argument to specify in advance the number of characters to print.
For details of this function, refer to the TextParameter class setWidth method and the TextWidthParameter class API reference.
4 To print the field data of array elements, specify the template area to repeat.
First, call the setEnableArrayFieldData method of the TemplateExtensionParameter class by passing true as the argument.
Next, call the setTemplateExtension method of the PrinterParameter class by passing the TemplateExtensionParameter instance to the method.
Then, call the PrinterParameter instance by passing it to the PrinterBuilder constructor.
This API call for PrinterBuilder is repeated as many times as the number of array elements specified in Step 5.
.add( PrinterBuilder( PrinterParameter() .setTemplateExtension( TemplateExtensionParameter() .setEnableArrayFieldData(true) ) )
5 To print the field data of array elements, call the actionPrintText method by passing a character string containing replacing specifiers that specify the field data of array elements (item_list key values).
.actionPrintText( "\${item_list.quantity}", TextParameter() .setWidth(2) ) .actionPrintText( "\${item_list.name}", TextParameter() .setWidth(24) ) .actionPrintText( "\${item_list.unit_price%6.2lf}\n", TextParameter() .setWidth(6) )
6 To print the numeric-type subtotal key value (8.24) in a specified format, call the actionPrintText method by passing a character string containing replacing specifiers that specify the number format.
.actionPrintText( "\${tax%6.2lf}\n", TextParameter() .setWidth( 6, TextWidthParameter() .setEllipsizeType(TextEllipsizeType.End) ) )
7 To print the barcode of the transaction_id key value ("0123456789"), call the actionPrintBarcode method by passing a character string containing a replacing specifier.
.actionPrintBarcode( BarcodeParameter("\${transaction_id}", BarcodeSymbology.Code128) .setPrintHri(true) )
8 To obtain the template, call the getCommands method.
val commands = builder.getCommands()
9 Prepare field data in JSON format. The texts in the specified areas in the template are replaced with this field data.
For how to send field data to a printer using the template created in this section, refer to Step 2.
Sample code
Field data
{
"store_name" : " Star Cafe ",
"order_number" : "#2-007",
"time" : "10/16 11:13PM",
"sales_type" : "Walk-in",
"server" : "Jane",
"transaction_id" : "0123456789",
"item_list" : [
{
"name" : "Vanilla Latte",
"unit_price" : 4.99,
"quantity" : 1
},
{
"name" : "Chocolate Chip Cookie",
"unit_price" : 3.25,
"quantity" : 1
}
],
"subtotal" : 8.24,
"tax" : 0.73,
"total" : 8.97,
"credit_card_number" : "VISA 0123",
"approval_code" : "OK2443",
"amount" : 8.97,
"address" : "123 Star Road, City,\nState 12345",
"tel" : "123-456-7890",
"mail" : "info@star-m.jp",
"url" : "star-m.jp"
}
Template Creation Process
builder.addDocument(
DocumentBuilder()
.settingPrintableArea(48.0)
.addPrinter(
PrinterBuilder()
.actionPrintImage(ImageParameter(logo, 406))
.styleInternationalCharacter(InternationalCharacterType.Usa)
.styleCharacterSpace(0.0)
.add(
PrinterBuilder()
.styleAlignment(Alignment.Center)
.styleBold(true)
.styleInvert(true)
.styleMagnification(MagnificationParameter(2, 2))
.actionPrintText("\${store_name}\n")
)
.actionFeed(1.0)
.actionPrintText(
"Order \${order_number}",
TextParameter()
.setWidth(
16
)
)
.actionPrintText(" ")
.actionPrintText(
"\${time}\n",
TextParameter()
.setWidth(
15,
TextWidthParameter()
.setAlignment(TextAlignment.Right)
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintText(
"Sale for \${sales_type}",
TextParameter()
.setWidth(16)
)
.actionPrintText(" ")
.actionPrintText(
"Served by \${server}\n",
TextParameter()
.setWidth(
15,
TextWidthParameter()
.setAlignment(TextAlignment.Right)
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintText(
"Transaction #\${transaction_id}\n"
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.add(
PrinterBuilder(
PrinterParameter()
.setTemplateExtension(
TemplateExtensionParameter()
.setEnableArrayFieldData(true)
)
)
.actionPrintText(
"\${item_list.quantity}",
TextParameter()
.setWidth(2)
)
.actionPrintText(
"\${item_list.name}",
TextParameter()
.setWidth(24)
)
.actionPrintText(
"\${item_list.unit_price%6.2lf}\n",
TextParameter()
.setWidth(6)
)
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.actionPrintText(
"Subtotal",
TextParameter()
.setWidth(26)
)
.actionPrintText(
"\${subtotal%6.2lf}\n",
TextParameter()
.setWidth(
6,
TextWidthParameter()
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintText(
"Tax",
TextParameter()
.setWidth(26)
)
.actionPrintText(
"\${tax%6.2lf}\n",
TextParameter()
.setWidth(
6,
TextWidthParameter()
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.actionPrintText(
"\${credit_card_number}",
TextParameter()
.setWidth(26)
)
.actionPrintText(
"\${total%6.2lf}\n",
TextParameter()
.setWidth(
6,
TextWidthParameter()
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintText(
"Approval Code",
TextParameter()
.setWidth(16)
)
.actionPrintText(
"\${approval_code}\n",
TextParameter()
.setWidth(
16,
TextWidthParameter()
.setAlignment(TextAlignment.Right)
)
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.actionPrintText(
"Amount",
TextParameter()
.setWidth(26)
)
.actionPrintText(
"\${amount%6.2lf}\n",
TextParameter()
.setWidth(
6,
TextWidthParameter()
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintText(
"Total",
TextParameter()
.setWidth(26)
)
.actionPrintText(
"\${total%6.2lf}\n",
TextParameter()
.setWidth(
6,
TextWidthParameter()
.setEllipsizeType(TextEllipsizeType.End)
)
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.actionPrintText(
"Signature\n"
)
.add(
PrinterBuilder()
.styleAlignment(Alignment.Center)
.actionPrintImage(
ImageParameter(signature, 256)
)
)
.actionPrintRuledLine(
RuledLineParameter(32.0)
.setX(8.0)
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.actionPrintText("\n")
.styleAlignment(Alignment.Center)
.actionPrintText(
"\${address}\n"
)
.actionPrintText(
"\${tel}\n"
)
.actionPrintText(
"\${mail}\n"
)
.actionFeed(1.0)
.actionPrintText(
"\${url}\n"
)
.actionPrintRuledLine(
RuledLineParameter(48.0)
)
.actionFeed(2.0)
.actionPrintText(
"Powered by Star Micronics\n"
)
.actionPrintBarcode(
BarcodeParameter("\${transaction_id}", BarcodeSymbology.Code128)
.setPrintHri(true)
)
.actionCut(CutType.Partial)
)
)
Template and Field Data Specifications
Template
A template is a print layout defined by a combination of fixed printing data and replacing specifiers, created with StarXpandCommandBuilder.
You can define a field (replacement area) in the template using a replacing specifier.
A value with the same key from field data in JSON format can be substituted for a replacing specifier.
As shown in the example below, you can insert a replacing specifier in a character string (content argument), such as the PrinterBuilder.actionPrintText method. A replacing specifier can coexist with a normal text.
StarXpandCommand.PrinterBuilder() .actionPrintText( "Date:${date} Time:${time}\n" )
PrinterBuilder() .actionPrintText( "Date:\${date} Time:\${time}\n" )
replacing specifier format
A key character string enclosed between ${ and }, like "${key-string}," is treated as a replacing specifier.
If there is a key that matches 'key-string' in the field data, the text in the "${key-string}" area is replaced with the key value.
If there is no matching key, printing is conducted with the "${key-string}" area ignored.

Key specifier
You can specify a key in the field data to identify the field data with which you want to replace.
Only ASCII characters are allowed in keys.
Case of a nested element
To specify a nested element, combine keys with a period (.).
Example of specifying a nested element
"${store.name}"
"\${store.name}"
Field data
{ "store" : { "name" : "Star Store", "address" : "123 Star Road, City, State 12345" } }
Case of an array element
By specifying an array element as the key for use with the key specifier, you can perform replacements for a single field based on the number of elements in the array.
In this case, you also need to define the range of the template to be repeated for the number of array elements. By passing a PrinterParameter/PageModeParameter embedded with a TemplateExtensionParameter (which enables array field data usage) to the PrinterBuilder/PageModeBuilder constructor, the corresponding PrinterBuilder/PageModeBuilder in the template will be repeated based on the number of elements in the array.
In the example below, keys of child elements of an array element (character string type and numeric type) are specified using key specifiers, and the repetition of array elements is specified by TemplateExtensionParameter. As a result, printing is executed only four times, the number of array elements in the field data, based on the PrinterBuilder repeat setting.
Template (partially omitted)
StarXpandCommand.PrinterBuilder() .add( // Repeated PrinterBuilder as many times as the number of array elements. StarXpandCommand.PrinterBuilder( StarXpandCommand.Printer.PrinterParameter() .setTemplateExtension( StarXpandCommand.TemplateExtensionParameter() .setEnableArrayFieldData(true) ) ) .actionPrintText( "${item_list.name}\t${item_list.unit_price%6.2lf}\n" ) .add( StarXpandCommand.PrinterBuilder() .styleUnderLine(true) .actionPrintText("\t\tx${item_list.quantity}\n") ) )
PrinterBuilder() .add( // Repeated PrinterBuilder as many times as the number of array elements. PrinterBuilder( PrinterParameter() .setTemplateExtension( TemplateExtensionParameter() .setEnableArrayFieldData(true) ) ) .actionPrintText( "\${item_list.name}\t\${item_list.unit_price%6.2lf}\n" ) .add( PrinterBuilder() .styleUnderLine(true) .actionPrintText("\t\tx\${item_list.quantity}\n") ) )
Field data
{ "item_list" : [ { "name" : "BLT", "unit_price" : 13.0, "quantity" : 1 }, { "name" : "Chicken Noodle", "unit_price" : 7.5, "quantity" : 1 }, { "name" : "Caesar salad", "unit_price" : 10.0, "quantity" : 2 }, { "name" : "Coffee", "unit_price" : 3.5, "quantity" : 2 } ] }
Printing result
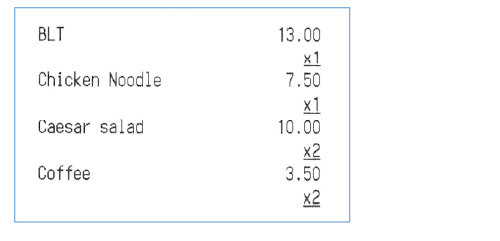
Number format specifier
If a field data value is a numeric type, you can specify the display format using a number format specifier. A number format specifier is a subset of a format specifier in printf function in C language. A number format specifier can be omitted. If the format specifier is omitted, the value is shown in the same format as when %d (outputs a signed integer in decimal) is specified.

Option | Meaning |
---|---|
♯ | Converts to another format (Adds 0x at the beginning for a hexadecimal value). |
0 | Fills with zeros. |
SP (space character) | Adds a space character to the left if the value is positive in a signed conversion. |
+ | Adds a plus sign if the value is positive. |
− | Aligns to the left. |
Number of displayed digits | [Total number of display digits].[Number of decimal places] Example: 8.3 (Eight digits overall, of which three digits are allocated after the decimal point.) |
Output format specifier | Meaning |
---|---|
d | Outputs a signed integer in decimal. |
u | Outputs an unsigned integer in decimal. |
f | Outputs a real number. |
x | Outputs an integer in lower-case hexadecimal. |
X | Outputs an integer in upper-case hexadecimal. |
ld | Outputs a signed long integer in decimal. |
lu | Outputs an unsigned long integer in decimal. |
lf | Outputs a double-precision real number. |
lx | Outputs a long integer in lower-case hexadecimal. |
lX | Outputs a long integer in upper-case hexadecimal. |
Escape characters
When you want to print ${ signifying the start of a replacing specifier, add a ; to the end for escape (${;).
To include the following characters, which have special meanings for the template printing function, in a field data key, when using them in a key specifier, place a \ before the characters for escape.
$ % . , }
APIs available for the template printing function
You can define a field using a replacing specifier in the following APIs.
Class | Method |
---|---|
PrinterBuilder | actionPrintText |
actionPrintBarcode | |
actionPrintPdf417 | |
actionPrintQRCode | |
PageModeBuilder | actionPrintText |
actionPrintBarcode | |
actionPrintPdf417 | |
actionPrintQRCode | |
DisplayBuilder | actionShowText(*) |
* Array elements cannot be specified.
Field data
Field data is a printing data set different for each printing, written in JSON format. The template printing function creates printing data by substituting the value of field data specified in a replacing specifier in a template.
Key specifications
Only ASCII characters are allowed in keys.
Value specifications
Which type of character string replaces the field is determined based on the field data value type as shown below.
JSON value type | Character string to replace with |
---|---|
Character string type | A value string |
Numeric type | A character string according to the number format specifier |
Null type | Does not print if the value in the key specifier is a null type. |
Boolean type | Prints the string "true" if the value is true or "false" if it is false. |
Object type | Does not print if the value of the key specifier is an object type. |
Array type | Complies with "Case of an array element" under "Key specifier.” |