Step 1 Generate Printing Data: Standard Function
Use PrinterBuilder to generate printing data.
PrinterBuilder provides you with useful methods to create printing data of receipts or labels.
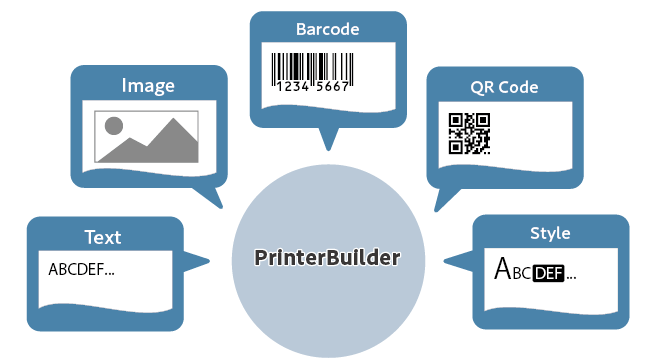
The following is a printing result of a print sample available in the SDK. The procedure below describes the areas indicated by the numbers.
Created printing data will be used in Step 2.
Create printing data using the procedure below.
The sample code is available at the bottom of the page.
Also, refer to the sample application in the SDK.
1 Generate the StarXpandCommandBuilder instance, which is used to generate printing data.
Call the addDocument method to add DocumentBuilder, and then call the addPrinter method to add PrinterBuilder.
Call the PrinterBuilder methods to generate printing data.
let builder = StarXpandCommand.StarXpandCommandBuilder() _ = builder.addDocument(StarXpandCommand.DocumentBuilder.init() .addPrinter(StarXpandCommand.PrinterBuilder()
2 Call the actionPrintImage method to print the image.
.actionPrintImage(StarXpandCommand.Printer.ImageParameter(image: logo, width: 406))
3 Call the styleInternationalCharacter method to set the international character set to USA.
.styleInternationalCharacter(.usa)
4 Call the styleCharacterSpace method to set the character spacing to 0 dots.
.styleCharacterSpace(0)
5 Call the styleAlignment method to set the alignment to the center.
.styleAlignment(.center)
6 Call the actionPrintText method to instruct the printing of the character string.
.actionPrintText("Star Clothing Boutique\n" + "123 Star Road\n" + "City, State 12345\n" + "\n")
Memo
TSP100III series and TSP100IIU+ do not support actionPrintText because these products are graphics-only printers.
Please use the actionPrintImage method to create printing data for these products. For other available methods, please also refer to "Supported Models" of each method.
7 Call the add method to add a nested PrinterBuilder instance.
Character decoration set in a nested PrinterBuilder is valid only in that PrinterBuilder, and cannot be transferred to the parent PrinterBuilder.
Call the styleMagnification method to set the double height and width expansion for the character string.
.add( StarXpandCommand.PrinterBuilder() .styleMagnification(StarXpandCommand.MagnificationParameter(width: 2, height: 2)) .actionPrintText(" $156.95\n") )
8 Call the styleInvert method to set black and white inversion for the character string.
.styleInvert(true)
9 Call the actionPrintBarcode method to instruct the printing of the barcode. You can set the barcode in BarcodeParameter.
.actionPrintBarcode(StarXpandCommand.Printer.BarcodeParameter(content: "0123456", symbology: .jan8) .setBarDots(3) .setHeight(5) .setPrintHRI(true))
10 Call the actionFeedLine method to instruct the paper feeding of one line of data.
.actionFeedLine(1)
11 Call the actionPrintQRCode method to instruct the printing of the QR code. You can set the QR code in QRCodeParameter.
.actionPrintQRCode(StarXpandCommand.Printer.QRCodeParameter(content: "Hello World.\n") .setLevel(.l) .setCellSize(8))
12 Call the actionCut method to instruct the paper cut while leaving one central point.
.actionCut(StarXpandCommand.Printer.CutType.partial)))
13 Call the getCommands method to acquire the printing data.
let command = builder.getCommands()
Sample code
let builder = StarXpandCommand.StarXpandCommandBuilder()
_ = builder.addDocument(StarXpandCommand.DocumentBuilder.init()
.addPrinter(StarXpandCommand.PrinterBuilder()
.actionPrintImage(StarXpandCommand.Printer.ImageParameter(image: logo, width: 406))
.styleInternationalCharacter(.usa)
.styleCharacterSpace(0)
.styleAlignment(.center)
.actionPrintText("Star Clothing Boutique\n" +
"123 Star Road\n" +
"City, State 12345\n" +
"\n")
.styleAlignment(.left)
.actionPrintText("Date:MM/DD/YYYY Time:HH:MM PM\n" +
"--------------------------------\n" +
"\n")
.add(
StarXpandCommand.PrinterBuilder()
.styleBold(true)
.actionPrintText("SALE \n")
)
.actionPrintText("SKU Description Total\n" +
"300678566 PLAIN T-SHIRT 10.99\n" +
"300692003 BLACK DENIM 29.99\n" +
"300651148 BLUE DENIM 29.99\n" +
"300642980 STRIPED DRESS 49.99\n" +
"300638471 BLACK BOOTS 35.99\n" +
"\n" +
"Subtotal 156.95\n" +
"Tax 0.00\n" +
"--------------------------------\n")
.actionPrintText("Total ")
.add(
StarXpandCommand.PrinterBuilder()
.styleMagnification(StarXpandCommand.MagnificationParameter(width: 2, height: 2))
.actionPrintText(" $156.95\n")
)
.actionPrintText("--------------------------------\n" +
"\n" +
"Charge\n" +
"156.95\n" +
"Visa XXXX-XXXX-XXXX-0123\n" +
"\n")
.add(
StarXpandCommand.PrinterBuilder()
.styleInvert(true)
.actionPrintText("Refunds and Exchanges\n")
)
.actionPrintText("Within ")
.add(
StarXpandCommand.PrinterBuilder()
.styleUnderLine(true)
.actionPrintText("30 days")
)
.actionPrintText(" with receipt\n" +
"And tags attached\n" +
"\n")
.styleAlignment(.center)
.actionPrintBarcode(StarXpandCommand.Printer.BarcodeParameter(content: "0123456", symbology: .jan8)
.setBarDots(3)
.setHeight(5)
.setPrintHRI(true))
.actionFeedLine(1)
.actionPrintQRCode(StarXpandCommand.Printer.QRCodeParameter(content: "Hello World.\n")
.setLevel(.l)
.setCellSize(8))
.actionCut(StarXpandCommand.Printer.CutType.partial)))
let command = builder.getCommands()
Use PrinterBuilder to generate printing data.
PrinterBuilder provides you with useful methods to create printing data of receipts or labels.
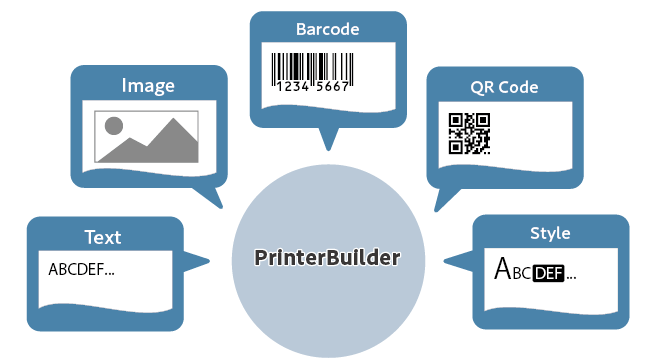
The following is a printing result of a print sample available in the SDK. The procedure below describes the areas indicated by the numbers.
Created printing data will be used in Step 2.
Create printing data using the procedure below.
The sample code is available at the bottom of the page.
Also, refer to the sample application in the SDK.
1 Generate the StarXpandCommandBuilder instance, which is used to generate printing data.
Call the addDocument method to add DocumentBuilder, and then call the addPrinter method to add PrinterBuilder.
Call the PrinterBuilder methods to generate printing data.
val builder = StarXpandCommandBuilder() builder.addDocument( DocumentBuilder() .addPrinter( PrinterBuilder()
2 Call the actionPrintImage method to instruct the printing of the image.
.actionPrintImage(ImageParameter(logo, 406))
3 Call the styleInternationalCharacter method to set the international character set to USB.
.styleInternationalCharacter(InternationalCharacterType.Usa)
4 Call the styleCharacterSpace method to set the character spacing to 0 dots.
.styleCharacterSpace(0.0)
5 Call the styleAlignment method to set the alignment to center.
.styleAlignment(Alignment.Center)
6 Call the actionPrintText method to instruct the printing of the character string.
.actionPrintText( "Star Clothing Boutique\n" + "123 Star Road\n" + "City, State 12345\n" + "\n" )
Memo
TSP100III series and TSP100IIU+ do not support actionPrintText because these products are graphics-only printers.
Please use the actionPrintImage method to create printing data for these products. For other available methods, please also refer to "Supported Models" of each method.
7 Call the add method so that you can add a nested PrinterBuilder instance.
Character decoration set in a nested PrinterBuilder is valid only in that PrinterBuilder but not transferred to the parent PrinterBuilder.
Call the styleMagnification method to set the double height and width expansion for the character string.
.add( PrinterBuilder() .styleMagnification(MagnificationParameter(2, 2)) .actionPrintText(" $156.95\n") )
8 Call the styleInvert method to set black and white inversion for the character string.
.styleInvert(true)
9 Call the actionPrintBarcode method to instruct the printing of the barcode. You can set the barcode in BarcodeParameter.
.actionPrintBarcode( BarcodeParameter("0123456", BarcodeSymbology.Jan8) .setBarDots(3) .setHeight(5.0) .setPrintHri(true) )
10 Call the actionFeedLine method to instruct the paper feeding of one line of data.
.actionFeedLine(1)
11 Call the actionPrintQRCode method to instruct the printing of the QR code. You can set the QR code in QRCodeParameter.
.actionPrintQRCode( QRCodeParameter("Hello, World\n") .setLevel(QRCodeLevel.L) .setCellSize(8) )
12 Call the actionCut method to instruct the paper cut while leaving one central point.
.actionCut(CutType.Partial) ) )
13 Call the getCommands method to acquire the printing data.
val commands = builder.getCommands()
Sample code
val builder = StarXpandCommandBuilder()
builder.addDocument(
DocumentBuilder()
.addPrinter(
PrinterBuilder()
.actionPrintImage(ImageParameter(logo, 406))
.styleInternationalCharacter(InternationalCharacterType.Usa)
.styleCharacterSpace(0.0)
.styleAlignment(Alignment.Center)
.actionPrintText(
"Star Clothing Boutique\n" +
"123 Star Road\n" +
"City, State 12345\n" +
"\n"
)
.styleAlignment(Alignment.Left)
.actionPrintText(
"Date:MM/DD/YYYY Time:HH:MM PM\n" +
"--------------------------------\n" +
"\n"
)
.add(
PrinterBuilder()
.styleBold(true)
.actionPrintText("SALE\n")
)
.actionPrintText(
"SKU Description Total\n" +
"300678566 PLAIN T-SHIRT 10.99\n" +
"300692003 BLACK DENIM 29.99\n" +
"300651148 BLUE DENIM 29.99\n" +
"300642980 STRIPED DRESS 49.99\n" +
"300638471 BLACK BOOTS 35.99\n" +
"\n" +
"Subtotal 156.95\n" +
"Tax 0.00\n" +
"--------------------------------\n"
)
.actionPrintText("Total ")
.add(
PrinterBuilder()
.styleMagnification(MagnificationParameter(2, 2))
.actionPrintText(" $156.95\n")
)
.actionPrintText(
"--------------------------------\n" +
"\n" +
"Charge\n" +
"156.95\n" +
"Visa XXXX-XXXX-XXXX-0123\n" +
"\n"
)
.add(
PrinterBuilder()
.styleInvert(true)
.actionPrintText("Refunds and Exchanges\n")
)
.actionPrintText("Within ")
.add(
PrinterBuilder()
.styleUnderLine(true)
.actionPrintText("30 days")
)
.actionPrintText(" with receipt\n")
.actionPrintText(
"And tags attached\n" +
"\n"
)
.styleAlignment(Alignment.Center)
.actionPrintBarcode(
BarcodeParameter("0123456", BarcodeSymbology.Jan8)
.setBarDots(3)
.setHeight(5.0)
.setPrintHri(true)
)
.actionFeedLine(1)
.actionPrintQRCode(
QRCodeParameter("Hello, World\n")
.setLevel(QRCodeLevel.L)
.setCellSize(8)
)
.actionCut(CutType.Partial)
)
)
val commands = builder.getCommands()