Step.1 印刷データの生成 : ページモード機能
ページモード機能は、あらかじめ指定したサイズの領域(ページ領域)内に位置指定をしながら文字や画像の描画を行うことができる機能です。
QRコードと同じ行内に文字列を配置する、横書きのレイアウトにするなど自由度の高いレイアウトの印刷データを生成できます。
ページモードの便利な3大機能
※項目にカーソルを合わせることで、イラストが変化します。
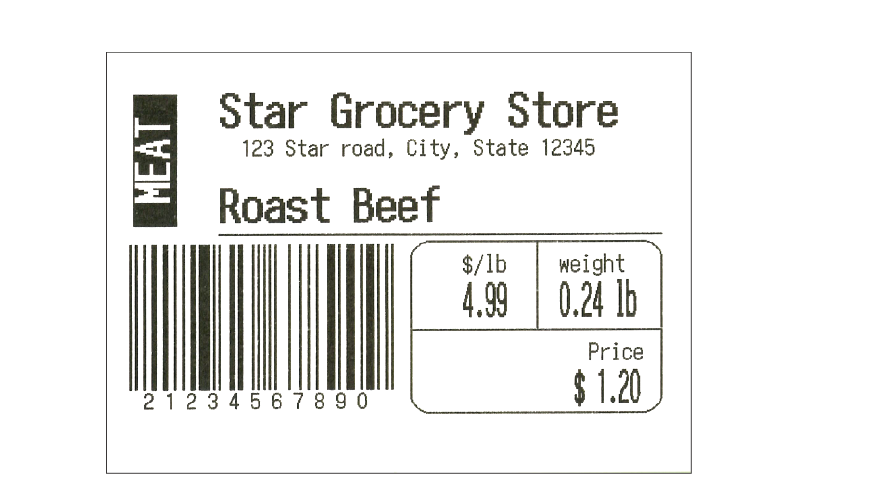
各印字要素を位置指定しながらレイアウトできます。例として、QRコードと同じ行内に文字列を配置することもできます。
2. 印字方向の設定
各印字要素の印字方向を指定しながらレイアウトできます。
3. 枠線・罫線の印字
枠線・罫線を印字することができます。罫線文字を使用するのに比べて長さや線幅などに細かい調整を行うことができます。
ページモード機能対応モデル
PrinterBuilderクラスのaddPageModeメソッドを使用できるモデルがページモード機能に対応しています。
対象モデルはリンク先にてご確認ください。
ページモード機能を利用した印刷データ生成処理の実装
PageModeBuilderを使って、ページモード機能を利用した印刷データを生成します。
1印刷データを生成するために使用するStarXpandCommandBuilderインスタンスを生成します。
ページモード機能を利用した印刷データの生成を行うためPrinterBuilderのaddPageModeメソッドを呼び出します。
最初の引数のPageModeAreaParameterでページ領域を指定します。
ここでは幅72mm、高さ30mmのページ領域を次の印字開始位置から3mm下の位置に設定します。
2番目の引数にPageModeBuilderインスタンスを渡します。
この後、ページモード機能を利用した印刷データを生成するため、PageModeBuilderの各メソッドを呼び出していきます。
var builder = new StarXpandCommand.StarXpandCommandBuilder(); builder.addDocument( new StarXpandCommand.DocumentBuilder() .addPrinter( new StarXpandCommand.PrinterBuilder() .addPageMode( new PageModeAreaParameter(72.0, 30.0), new StarXpandCommand.PageModeBuilder()
2四角形の枠線の印刷を指示するためactionPrintRectangleメソッドを呼び出します。
四角形の枠線の設定はPageModeRectangleParameterにて行います。
ここでは、ページ領域の左上から横方向に5mm、縦方向に2mmの位置に、幅62mm、高さ26mmの四角形(各頂点は半径5mmの角丸)の枠線を設定しています。
.actionPrintRectangle( new PageModeRectangleParameter(5.0, 2.0, 62.0, 26.0) .setRoundCorner(true) .setCornerRadius(5.0) )
3ページ領域左上から絶対位置にて次のQRコードの印字開始位置を指定するため、styleVerticalPositionToメソッド、styleHorizontalPositionToメソッドを呼び出します。
QRコードの印刷を指示するため、actionPrintQRCodeメソッドを呼び出します。QRコードの設定はQRCodeParameterにて行います。
.styleVerticalPositionTo(5.0) .styleHorizontalPositionTo(10.0) .actionPrintQRCode( new StarXpandCommand.Printer.QRCodeParameter('Hello World.\n') .setLevel(StarXpandCommand.Printer.QRCodeLevel.L) .setCellSize(6) )
4前の印字終了位置からの相対位置にて次の文字列の印字開始位置を指定するため、styleHorizontalPositionByメソッドを呼び出します。
.styleHorizontalPositionBy(10.0) .actionPrintText("ABC")
5これ以降の印字方向をTopToBottomに設定するため、 stylePrintDirectionメソッドを呼び出します。
.stylePrintDirection(PageModePrintDirection.TopToBottom)
6ページ領域右上から絶対位置にて次の文字列の印字開始位置を指定するため、styleVerticalPositionToメソッド、 styleHorizontalPositionToメソッドを呼び出します。
.styleVerticalPositionTo(10.0) .styleHorizontalPositionTo(10.0) .actionPrintText("EFG")
7中央一点残しの用紙カットを指示するため、actionCutメソッドを呼び出します。
.actionCut(CutType.Partial)
8印刷データを取得するためgetCommandsメソッドを呼び出します。
var commands = await builder.getCommands();
サンプルコード
var builder = new StarXpandCommand.StarXpandCommandBuilder();
builder.addDocument(
new StarXpandCommand.DocumentBuilder()
.addPrinter(
new StarXpandCommand.PrinterBuilder()
.addPageMode(
new PageModeAreaParameter(72.0, 30.0),
new StarXpandCommand.PageModeBuilder()
.actionPrintRectangle(
new PageModeRectangleParameter(5.0, 2.0, 62.0, 26.0)
.setRoundCorner(true)
.setCornerRadius(5.0)
)
.styleVerticalPositionTo(5.0)
.styleHorizontalPositionTo(10.0)
.actionPrintQRCode(
new StarXpandCommand.Printer.QRCodeParameter('Hello World.\n')
.setLevel(StarXpandCommand.Printer.QRCodeLevel.L)
.setCellSize(6)
)
.styleHorizontalPositionBy(10.0)
.actionPrintText("ABC")
.stylePrintDirection(PageModePrintDirection.TopToBottom)
.styleVerticalPositionTo(10.0)
.styleHorizontalPositionTo(10.0)
.actionPrintText("EFG")
)
.actionCut(CutType.Partial)
)
);
var commands = await builder.getCommands();