Step 2 Send Printing Data: Spooler Function
The spooler function makes print requests from upper level terminals always receivable by the printer and prints them in the order they are received.
The printer manages each print request as a job.
* With the conventional method, if a new print request is made before the previous printing is completed, the transmission of the request is held up or cause a communication error, and a new print request was not accepted until the previous printing completed.
Four Major Useful Features of Spooler Function
1. Multiple pieces of printing data can be sent before the printing is completed.
The printer can put print jobs from upper level terminals in the queue and print them in the order they are received. The print job can be sent to MCL32CI/MCL32CBI even when the taken paper sensor is detecting paper.
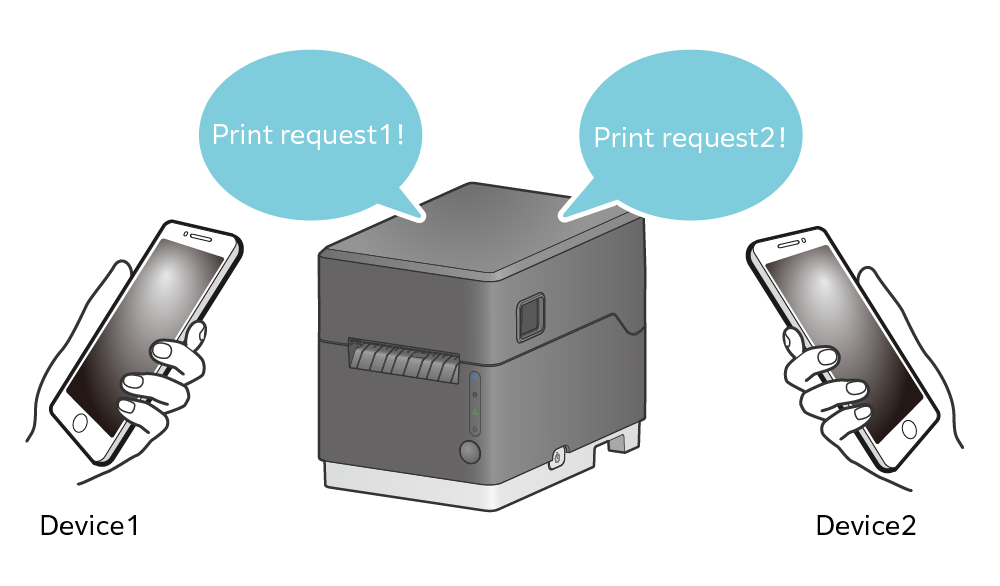
2. Multiple terminals can use the printer .
Even when print requests are made from multiple upper level terminals, they are received without a communication error.
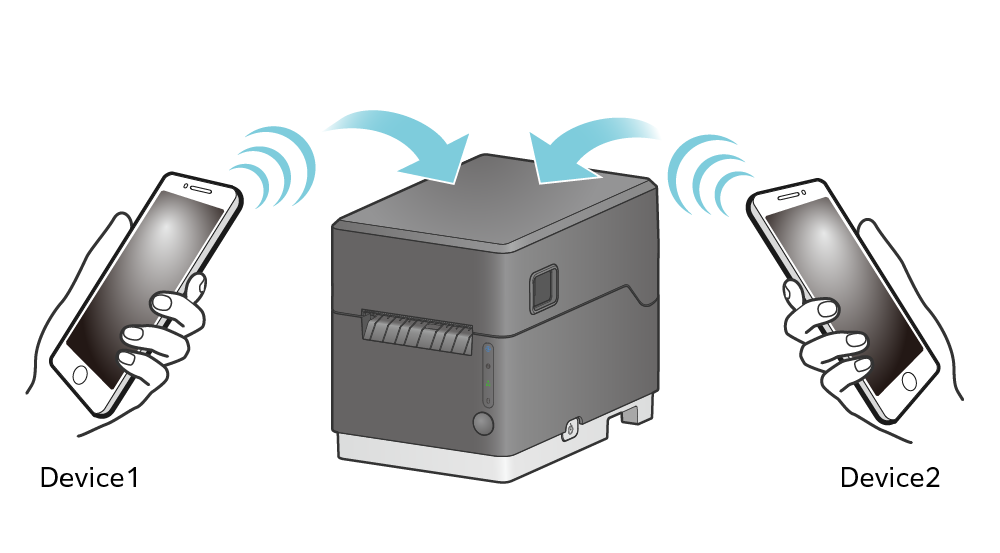
3. Automatic reprinting
Even if printing fails due to an error such as no paper, performing error recovery (replacing the paper in the case of no paper) allows automatic reprinting.
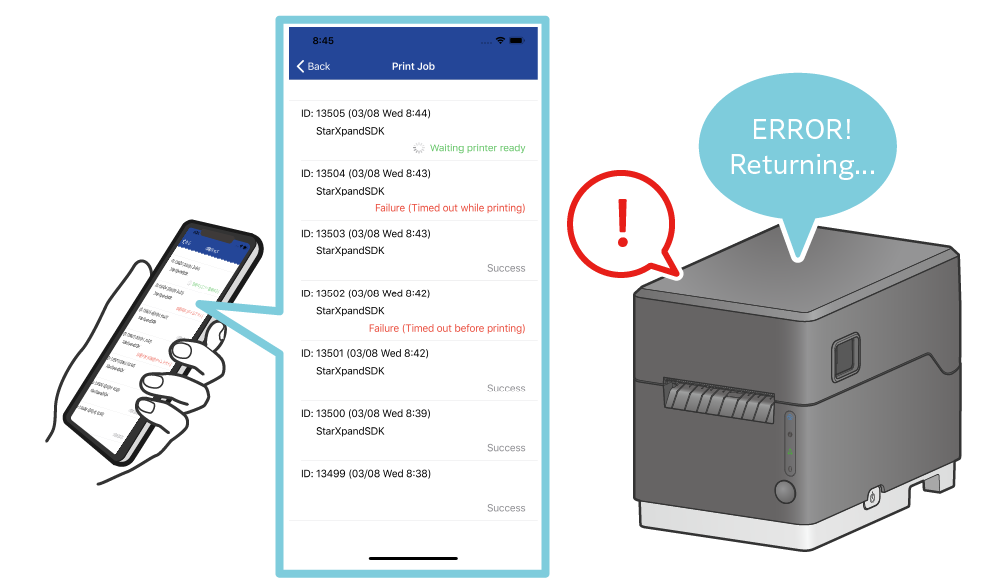
4. The status and history of print jobs can be checked anytime.
You can check print results (success and failure) and reception status.
Up to 2000 pieces of latest job information are available.
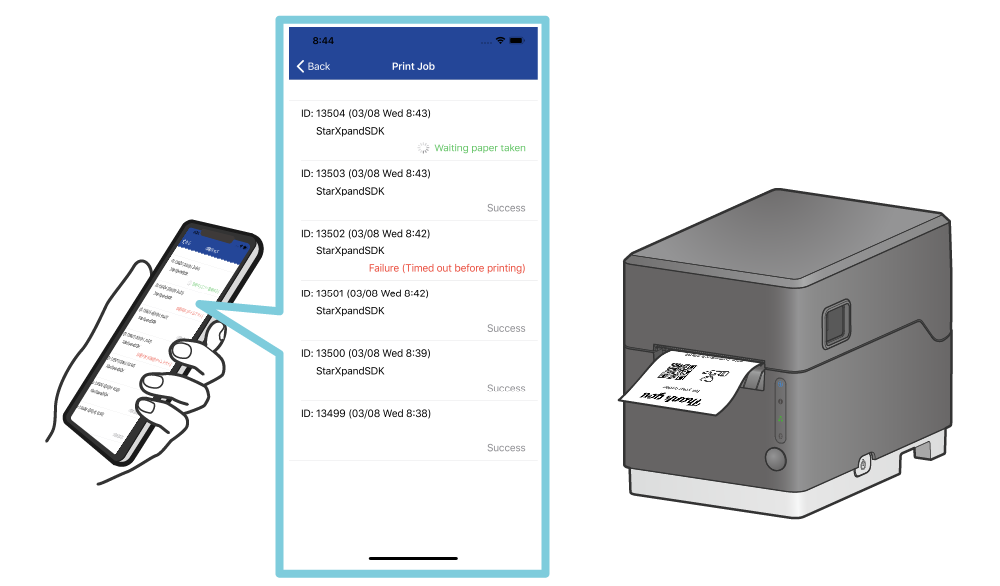
Note:
There are the following restrictions regarding simultaneously connecting multiple host devices or interfaces.
For details, see Precautions for Using Multi-interface.
To use the spooler function
To use the spooler function, set the printer's spooler function to enable. (Default: Disabled)
Also, use the print(command: string, jobSettings: StarSpoolJobSetttings) method, which includes print job settings, instead of the print(commad: string) method used to send print data in Sending Printing Data: Standard Function.
Other features include the ability to check the status of print jobs.
Please refer to the Implement Printing Process Using Spooler Function.
Spooler Function Supported Model
Models that allow the use of the print(command: string, jobSettings: StarSpoolJobSettings) method support the spooler function.
Please click this link to check supported model.
Implement Printing Process Using Spooler Function
You will send the printing data created in Step 1 to the printer and print it.
To use the spooler function by using the StarPrinter class, call the print(command: string, jobSettings: StarSpoolJobSetttings) method to send the printing data.
The following description is based on the sample code. Send the printing data using the procedure below.
The sample code is available at the bottom of the page.
Also, refer to the sample application in the SDK.
1 Create the printer destination information.
Set interfaceType, which is used for communication, and identifier, which specifies the destination.
If the destination printer has already been searched for in Search for Printer, this setting is unnecessary.
Set the StarPrinter instance acquired with the onPrinterFound method in the printer variable in Step2.
var settings = new StarConnectionSettings(); settings.interfaceType = InterfaceType.Lan; settings.identifier = “00:11:62:00:00:01”;
2 Provide the printer destination information to acquire the StarPrinter instance.
var printer = new StarPrinter(settings);
3 Set the printing data created in Step 1 in the commands variable.
try { var commands: string = ...
4 Use the StarSpoolJobSettings class to set the print job. In the following setting example, retry on printer error is enabled, print job timeout is 30 seconds, and information added to print jobs is set to "Print from Tablet1".
var jobSettings = StarSpoolJobSettings(true, 30, "Print from Tablet1");
5 Call the open method to connect to the printer.
await printer.open();
6 Call the print method to send the printing data to the printer. Acquire the job ID with the return value.
var jobId = await printer.print(commands, jobSettings);
7 There are the following two methods to check the print job status.
Call the getSpoolJobStatus method to acquire the status of the specified job ID.
var jobStatus = await printer.getSpoolJobStatus(jobId);
Call the getSpoolJobStatusList method to acquire the status list of as many jobs as specified in order of newest job received first.
If 10 is set in the argument, the status list of 10 jobs in order from newest to oldest is acquired.
var jobStatusList = await printer.getSpoolJobStatusList(10); }
8 Implement the process for when an error occurs. You can identify the error cause based on the error instance type. Possible errors are described in the API reference of the open method, print method, getSpoolJobStatus method, and getSpoolJobStatusList method of the StarPrinter class.
} catch(error) { if (error instanceof StarIO10UnprintableError) { if (error.codeCode == StarIO10ErrorCode.SpoolerIsDisabled) { // The spooler function is set to disabled. // Please set the printer's spooler function to enable. } } console.log(`Error: ${String(error)}`); }
9 Call the close method to disconnect from the printer.
finally { await printer.close();
10 Call the dispose method to dispose the printer instance.
await printer.dispose(); }
Sample code
var settings = new StarConnectionSettings();
settings.interfaceType = InterfaceType.Lan;
settings.identifier = "00:11:62:00:00:01";
var printer = new StarPrinter(settings);
try {
// Set the print data created in Step 1 to the commands variable.
var commands = ...
var jobSettings = StarSpoolJobSettings(true, 30, "Print from Tablet1");
await printer.open();
var jobId = await printer.print(commands, jobSettings);
var jobStatus = await printer.getSpoolJobStatus(jobId);
// var jobStatusList = await printer.getSpoolJobStatusList(10);
}
catch(error) {
if (error instanceof StarIO10UnprintableError) {
if (error.codeCode == StarIO10ErrorCode.SpoolerIsDisabled) {
// The spooler function is set to disabled.
// Please set the printer's spooler function to enable.
}
}
console.log(`Error: ${String(error)}`);
}
finally {
await printer.close();
await printer.dispose();
}
Spooler Function Sequence Figure
This is a sequence figure showing a process of checking the print jobs until all the printing is completed after two upper level terminals make print requests to one printer.